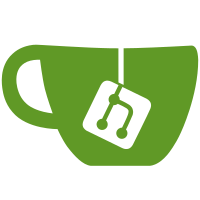
We're using the Gram matrix engine for the next stage of development, so the algebraic engine shouldn't be at the top level anymore.
76 lines
1.8 KiB
Julia
76 lines
1.8 KiB
Julia
include("HittingSet.jl")
|
|
|
|
module Engine
|
|
|
|
include("Engine.Algebraic.jl")
|
|
include("Engine.Numerical.jl")
|
|
|
|
using .Algebraic
|
|
using .Numerical
|
|
|
|
export Construction, mprod, codimension, dimension
|
|
|
|
end
|
|
|
|
# ~~~ sandbox setup ~~~
|
|
|
|
using Random
|
|
using Distributions
|
|
using LinearAlgebra
|
|
using AbstractAlgebra
|
|
using HomotopyContinuation
|
|
using GLMakie
|
|
|
|
CoeffType = Rational{Int64}
|
|
|
|
spheres = [Engine.Sphere{CoeffType}() for _ in 1:3]
|
|
tangencies = [
|
|
Engine.AlignsWithBy{CoeffType}(
|
|
spheres[n],
|
|
spheres[mod1(n+1, length(spheres))],
|
|
CoeffType(1)
|
|
)
|
|
for n in 1:3
|
|
]
|
|
ctx_tan_sph = Engine.Construction{CoeffType}(elements = spheres, relations = tangencies)
|
|
ideal_tan_sph, eqns_tan_sph = Engine.realize(ctx_tan_sph)
|
|
freedom = Engine.dimension(ideal_tan_sph)
|
|
println("Three mutually tangent spheres: $freedom degrees of freedom")
|
|
|
|
# --- test rational cut ---
|
|
|
|
coordring = base_ring(ideal_tan_sph)
|
|
vbls = Variable.(symbols(coordring))
|
|
|
|
# test a random witness set
|
|
system = CompiledSystem(System(eqns_tan_sph, variables = vbls))
|
|
norm2 = vec -> real(dot(conj.(vec), vec))
|
|
rng = MersenneTwister(6071)
|
|
n_planes = 6
|
|
samples = []
|
|
for _ in 1:n_planes
|
|
real_solns = solution.(Engine.Numerical.real_samples(system, freedom, rng = rng))
|
|
for soln in real_solns
|
|
if all(norm2(soln - samp) > 1e-4*length(gens(coordring)) for samp in samples)
|
|
push!(samples, soln)
|
|
end
|
|
end
|
|
end
|
|
println("Found $(length(samples)) sample solutions")
|
|
|
|
# show a sample solution
|
|
function show_solution(ctx, vals)
|
|
# evaluate elements
|
|
real_vals = real.(vals)
|
|
disp_points = [Engine.Numerical.evaluate(pt, real_vals) for pt in ctx.points]
|
|
disp_spheres = [Engine.Numerical.evaluate(sph, real_vals) for sph in ctx.spheres]
|
|
|
|
# create scene
|
|
scene = Scene()
|
|
cam3d!(scene)
|
|
scatter!(scene, disp_points, color = :green)
|
|
for sph in disp_spheres
|
|
mesh!(scene, sph, color = :gray)
|
|
end
|
|
scene
|
|
end |