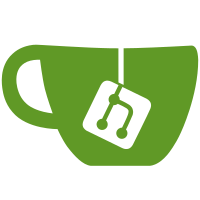
At the end of the realization routine, use the computed Hessian to find the tangent space of the solution variety, and return it alongside the realization. Since altering the constraints can change the tangent space without changing the solution, we compute the tangent space even when the guess passed to the realization routine is already a solution.
40 lines
1.3 KiB
Rust
40 lines
1.3 KiB
Rust
use nalgebra::DMatrix;
|
|
|
|
use dyna3::engine::{Q, realize_gram, sphere, PartialMatrix};
|
|
|
|
fn main() {
|
|
let gram = {
|
|
let mut gram_to_be = PartialMatrix::new();
|
|
for j in 0..3 {
|
|
for k in j..3 {
|
|
gram_to_be.push_sym(j, k, if j == k { 1.0 } else { -1.0 });
|
|
}
|
|
}
|
|
gram_to_be
|
|
};
|
|
let guess = {
|
|
let a: f64 = 0.75_f64.sqrt();
|
|
DMatrix::from_columns(&[
|
|
sphere(1.0, 0.0, 0.0, 1.0),
|
|
sphere(-0.5, a, 0.0, 1.0),
|
|
sphere(-0.5, -a, 0.0, 1.0)
|
|
])
|
|
};
|
|
println!();
|
|
let (config, _, success, history) = realize_gram(
|
|
&gram, guess, &[],
|
|
1.0e-12, 0.5, 0.9, 1.1, 200, 110
|
|
);
|
|
print!("\nCompleted Gram matrix:{}", config.tr_mul(&*Q) * &config);
|
|
if success {
|
|
println!("Target accuracy achieved!");
|
|
} else {
|
|
println!("Failed to reach target accuracy");
|
|
}
|
|
println!("Steps: {}", history.scaled_loss.len() - 1);
|
|
println!("Loss: {}", history.scaled_loss.last().unwrap());
|
|
println!("\nStep │ Loss\n─────┼────────────────────────────────");
|
|
for (step, scaled_loss) in history.scaled_loss.into_iter().enumerate() {
|
|
println!("{:<4} │ {}", step, scaled_loss);
|
|
}
|
|
} |