forked from StudioInfinity/dyna3
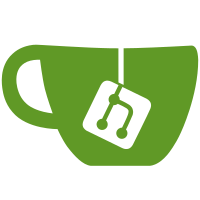
This will make it easier for elements and regulators to write themselves into the constraint problem.
31 lines
No EOL
1.1 KiB
Rust
31 lines
No EOL
1.1 KiB
Rust
use dyna3::engine::{Q, point, realize_gram, sphere, ConstraintProblem};
|
|
|
|
fn main() {
|
|
let mut problem = ConstraintProblem::from_guess(&[
|
|
point(0.0, 0.0, 2.0),
|
|
sphere(0.0, 0.0, 0.0, 1.0)
|
|
]);
|
|
for j in 0..2 {
|
|
for k in j..2 {
|
|
problem.gram.push_sym(j, k, if (j, k) == (1, 1) { 1.0 } else { 0.0 });
|
|
}
|
|
}
|
|
problem.frozen.push((3, 0));
|
|
println!();
|
|
let (config, _, success, history) = realize_gram(
|
|
&problem, 1.0e-12, 0.5, 0.9, 1.1, 200, 110
|
|
);
|
|
print!("\nCompleted Gram matrix:{}", config.tr_mul(&*Q) * &config);
|
|
print!("Configuration:{}", config);
|
|
if success {
|
|
println!("Target accuracy achieved!");
|
|
} else {
|
|
println!("Failed to reach target accuracy");
|
|
}
|
|
println!("Steps: {}", history.scaled_loss.len() - 1);
|
|
println!("Loss: {}", history.scaled_loss.last().unwrap());
|
|
println!("\nStep │ Loss\n─────┼────────────────────────────────");
|
|
for (step, scaled_loss) in history.scaled_loss.into_iter().enumerate() {
|
|
println!("{:<4} │ {}", step, scaled_loss);
|
|
}
|
|
} |